Creating a CRUD (Create, Read, Update, Delete) app might sound like a big task if you're new to web development. But don't worry! This guide will help you build one using Next.js and Supabase, step by step. With Next.js, a popular React framework, and Supabase, a backend service with a real-time database, you have everything you need to bring your app to life.
Imagine building your own mini web app where you can add, view, edit, and remove data. It might sound like magic, but it's more like assembling a Lego set. Each piece has its place, and by the end, you'll have something functional and fun. So, roll up your sleeves and let's dive into building a simple CRUD app that you can show off to your friends or even use as a base for your next big project.
Setting up your environment
Before we start coding, let's make sure you have everything set up. Preparing your environment will make the rest of the process much smoother.
Installing Node.js and Next.js
First, you need Node.js. It's like the engine that powers your app. You can download it from the official Node.js website. Once you have Node.js, installing Next.js is easy. Just open your terminal and type:
This command sets up a new Next.js project called "my-crud-app." You'll see a bunch of files and folders, but don't worry, we'll walk through them together.
Setting up Supabase
Now, let's set up Supabase. Go to the Supabase website and sign up if you haven't already. Create a new project, and you'll get a URL and an API key. These are your golden tickets to connect your app to Supabase. Keep them safe, like how you protect your Netflix password.
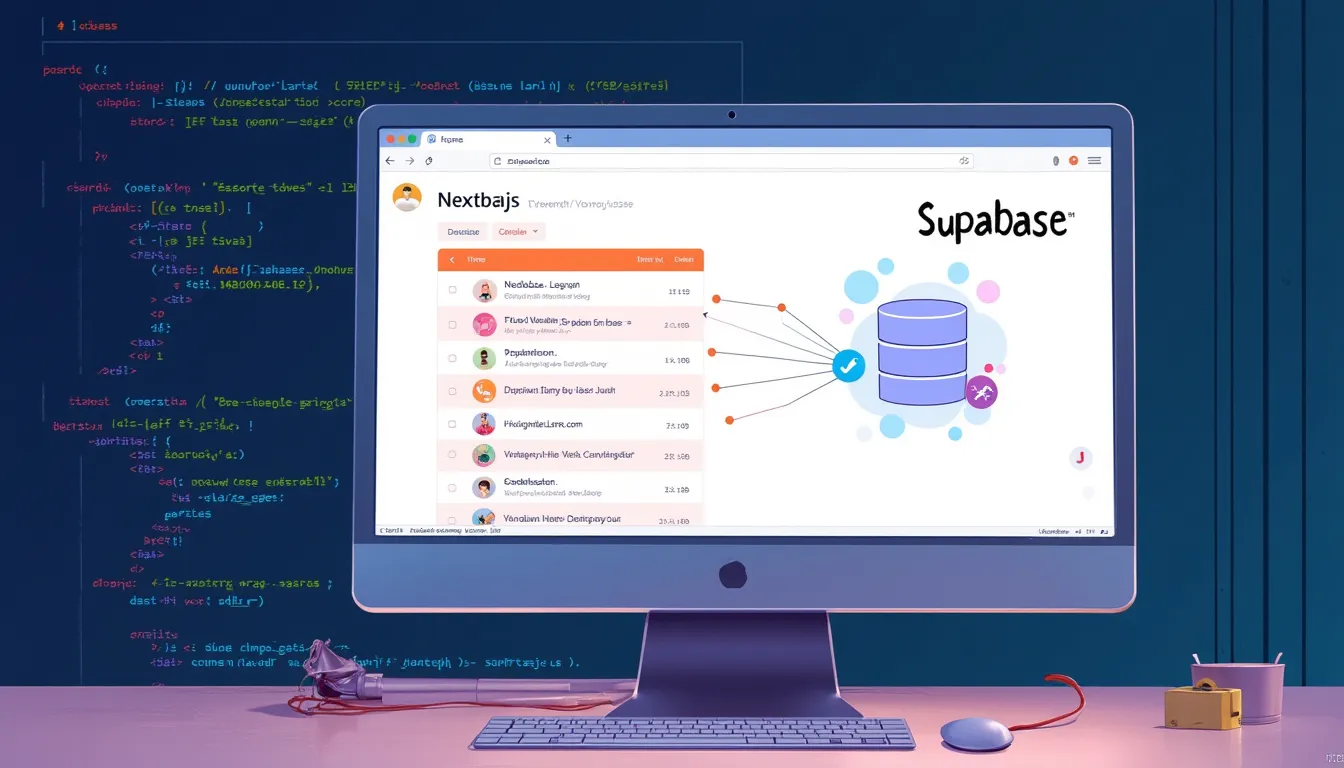
Building the CRUD app
With your environment ready, it's time for the fun part—building the app. We'll go through each of the CRUD operations one by one, so you see how everything fits together.
Creating data
Let's start by adding new data to our app. In a CRUD app, creating data is like adding a new item to a list. For example, if you're building a to-do list app, adding a task would be the 'create' part.
-
Open your Next.js project.
-
Import Supabase at the top of your file:
-
Initialize the Supabase client using your URL and API key:
-
Create a function to add data:
Reading data
Reading data is about fetching and displaying the information stored in your database. Using our to-do list example, this is like fetching the list of tasks to display on your screen.
-
Create a function to fetch data from your database:
-
Call this function to display data in your app.
Updating data
Updating data means changing existing data in your app. Imagine you completed a task on your to-do list and want to mark it as done.
- Create a function to update data:
Deleting data
Lastly, deleting data is like erasing a task from your to-do list when it's no longer needed.
- Create a function to delete data:
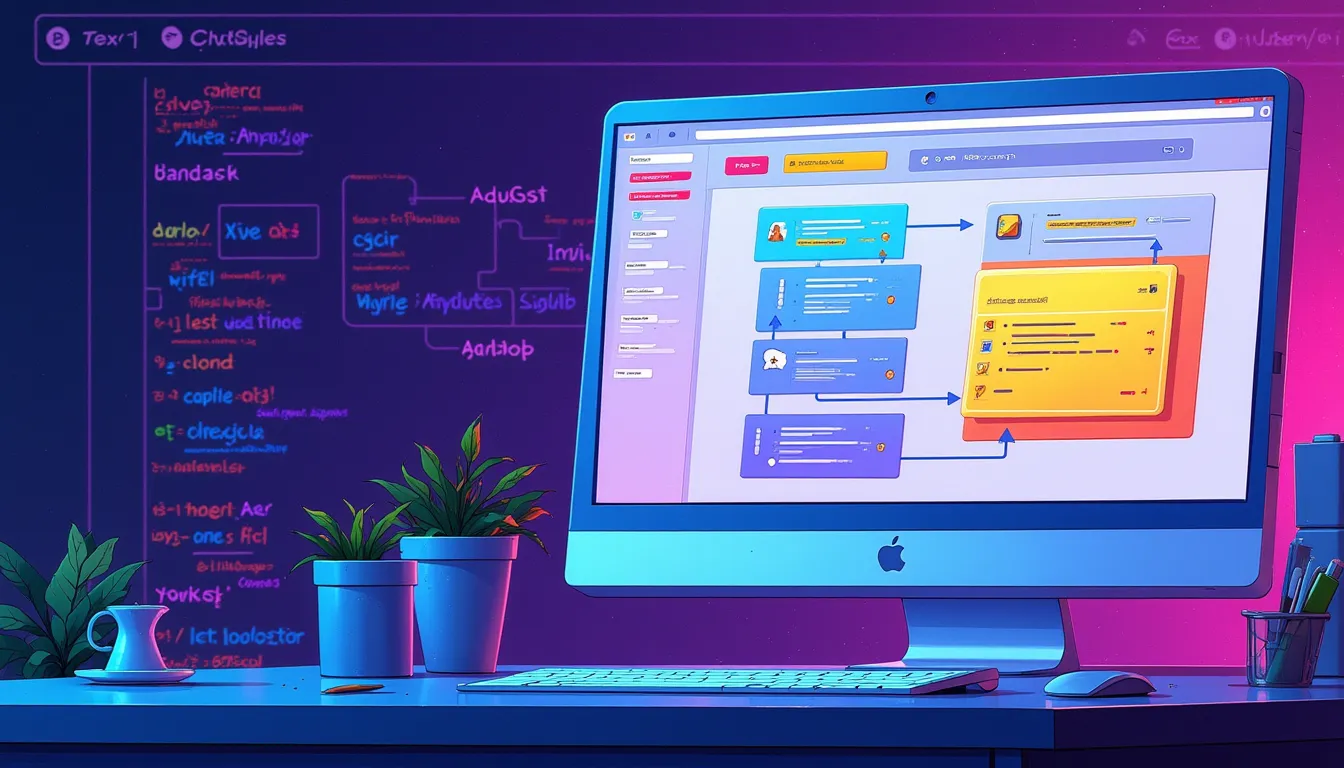
Testing and deploying
You've built your app, and it's time to test and deploy it. Testing ensures everything works as expected, and deploying lets you share your creation with the world.
Testing your CRUD app
Before deploying, run your app locally to check if everything works. Use the command below to start your Next.js development server:
Visit in your browser to see your app in action. Try adding, reading, updating, and deleting data to make sure everything functions correctly.
Deploying your app
Deploying your app is like sending it off to college—it's time for it to shine on its own. Platforms like Vercel make it easy to deploy Next.js apps. You can link your GitHub repository, and Vercel will handle the rest.
Conclusion
Congratulations! You've built a CRUD app using Next.js and Supabase. By following this guide, you should now understand how these two powerful tools can work together. Whether you're building a personal project or the next big thing, remember that every app starts with a simple idea and the willingness to build.
Feel free to customize your app and explore more features that Next.js and Supabase offer. The journey doesn't end here, and there's always more to learn and create. Happy coding!
FAQ
What is a CRUD app?
A CRUD app is a type of application that allows users to create, read, update, and delete data. It's a fundamental concept in web development and is used in various applications like to-do lists, inventory management systems, and more.
Why use Next.js for building a CRUD app?
Next.js is a popular React framework that offers server-side rendering, static site generation, and many other features that enhance performance and development efficiency. It is well-suited for building modern web applications, including CRUD apps.
How does Supabase help in building a CRUD app?
Supabase provides a real-time database and authentication services, making it easier to manage and interact with your app's data. It simplifies backend development, allowing you to focus more on building the front end of your application.
Can I deploy my Next.js CRUD app for free?
Yes, you can deploy your Next.js CRUD app for free using platforms like Vercel, which offers seamless integration with Next.js and GitHub, making deployment straightforward and cost-effective.
Is it necessary to have prior experience in web development to build a CRUD app?
While prior experience can be helpful, this guide is designed to be beginner-friendly. By following the step-by-step instructions, even those new to web development can successfully build a CRUD app.