Learn how to effectively use the useId function in React to enhance your Next.js apps. This guide breaks down the basics and shows you why and how to implement it smoothly.
In the world of web development, every little tool or function can make a big difference. Enter useId, a handy hook introduced in React 18. If you're working with Next.js projects, you might have stumbled upon this feature or heard whispers about it. But what is it, and why should you care? Well, buckle up, because we're about to dive into the world of unique identifiers in React and how they can enhance your apps.
Imagine you're at a party, and everyone is wearing the same outfit. Confusing, right? Now, picture they all have name tags. That's what useId does for your components. It gives them unique identifiers, making it easier to manage and distinguish them. This is especially useful in forms, lists, and any scenario involving dynamic elements.
If you're scratching your head wondering how to bring this magic into your Next.js projects, you're in the right place. We'll walk you through the basics, showcase examples, and share why this hook is a game-changer.
What is the useId hook?
The need for unique identifiers
In React development, components often need unique identifiers to maintain order and avoid chaos. Think of it like assigning each student a roll number in school. Without it, calling attendance would be a nightmare! In the digital space, these identifiers help in linking labels to inputs in forms, keeping track of elements in lists, and ensuring that dynamic content is rendered correctly.
How useId works
The useId hook provides a unique ID in the context of the component. It's like a little helper that makes sure each component can be uniquely identified. This is particularly useful when you're dealing with server rendering, where duplicate IDs can mess things up. With useId, you get:
- Stability between server and client
- Reduced risk of ID clashes
- Improved accessibility with proper label-input pairings
Here's a simple example to illustrate:
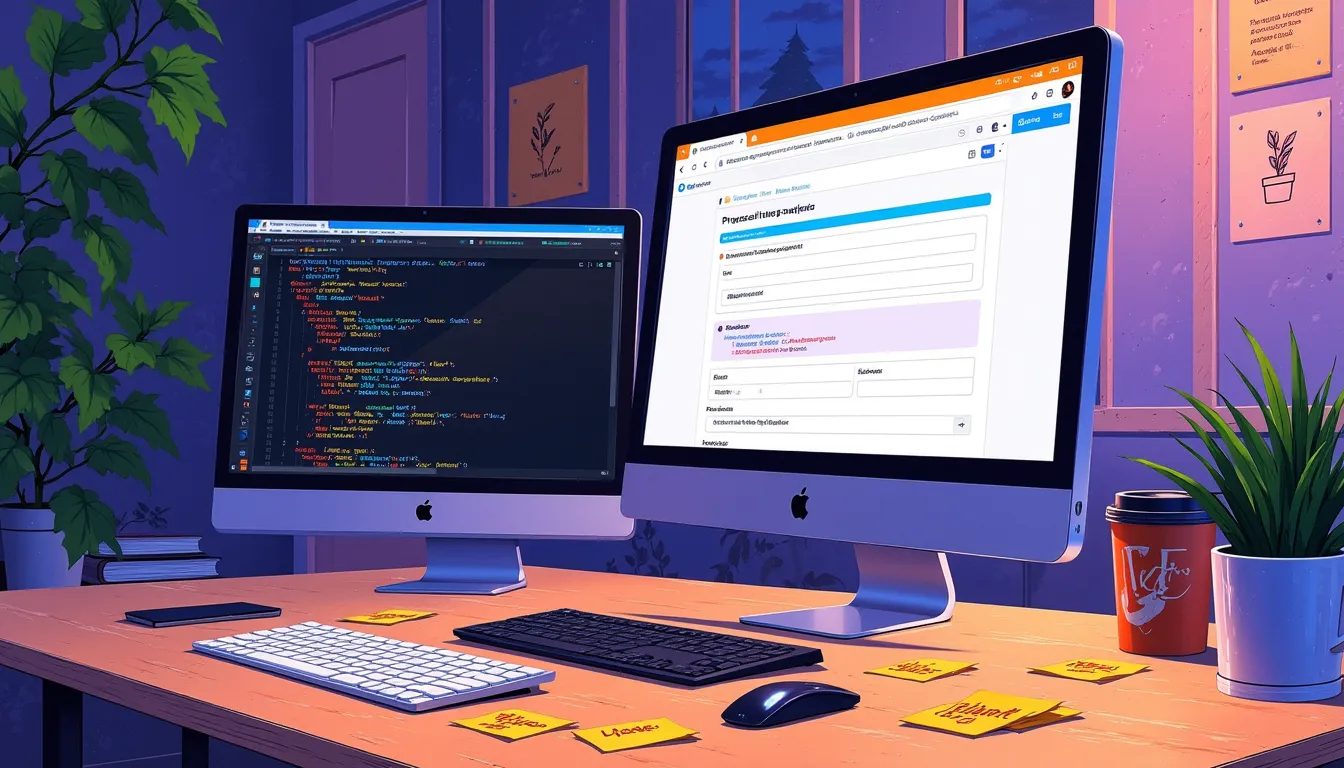
See how neat that is? The useId hook ensures that the label is correctly associated with the input, improving accessibility.
Implementing useId in your Next.js app
Setting up a Next.js project
Before we jump into using useId, let's make sure you have a Next.js project ready. If you're new to Next.js, don't worry, getting started is easy. Open your terminal and run:
This will set up a basic Next.js app and launch it on localhost. You're ready to start coding!
Using useId in components
Now that your Next.js app is up and running, let's see how useId can be integrated. Let's say you're building a form with multiple input fields. Each input needs a unique ID to ensure the labels are correctly linked.
Each input field gets a unique ID, ensuring no mix-up in their labels. It's as simple as that!
Why useId is a game-changer in Next.js
Server-side rendering benefits
One major win with useId is its compatibility with server-side rendering (SSR) in Next.js. Normally, rendering on the server and then rehydrating on the client can lead to mismatches in IDs. But with useId, you have consistent IDs across both environments. No more unexpected behavior or errors!
Enhancing accessibility
Accessibility is key in web development. With useId, you ensure that labels are properly linked to inputs, making your apps more accessible to users relying on screen readers. It's like giving your app a VIP pass to inclusivity.
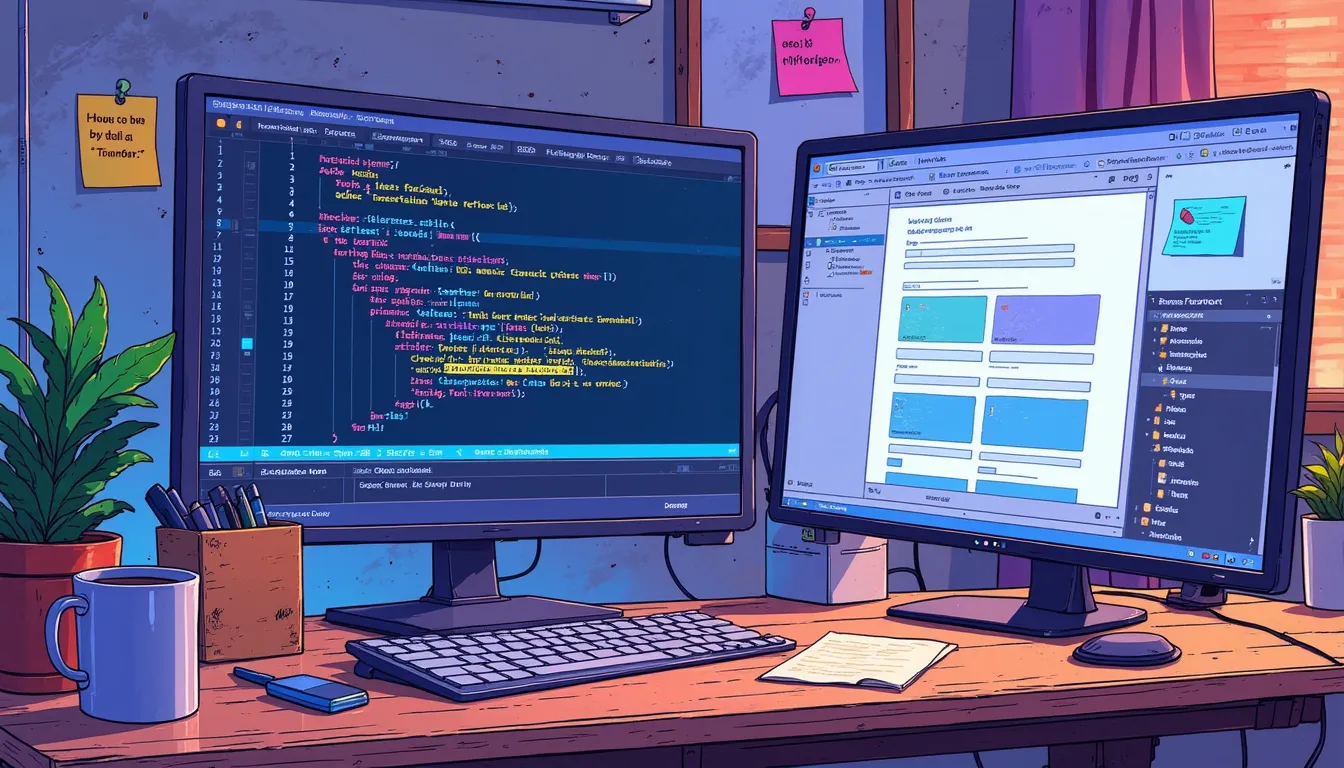
Best practices and tips
Keep it simple
While useId is a powerful tool, it's important to use it wisely. Not every component needs a unique ID. Reserve it for scenarios where IDs are essential, like forms or dynamic lists.
Combining with other hooks
Don't hesitate to combine useId with other React hooks. For example, you can use useState to manage form data alongside useId for IDs. This way, your components stay organized and efficient.
Stay updated
React is always evolving. Make sure you're using the latest version to get the most out of useId. You can always check the React documentation for updates and best practices.
Conclusion
And there you have it! A deep dive into useId and how it can enhance your Next.js projects. It's a simple yet effective tool that brings order to your components, ensures accessibility, and plays well with server-side rendering. By integrating useId, you're not just adding unique identifiers; you're enhancing the overall quality and reliability of your apps.
Whether you're building a small form or a large-scale application, useId is a hook worth having in your toolkit. So go ahead, give your components the unique IDs they deserve, and watch your projects flourish!
Happy coding!
FAQ
What is the primary use of the useId hook in React?
The primary use of the useId hook is to provide unique identifiers for components in a React application, ensuring stability between server and client, reducing the risk of ID clashes, and enhancing accessibility by linking labels to inputs properly.
Can useId be used in all React versions?
No, useId is available starting from React 18. Ensure your project is running on this version or higher to utilize this hook.
How does useId enhance accessibility in web applications?
useId enhances accessibility by ensuring that input fields in forms have unique IDs, which can be correctly paired with their respective labels, thereby improving the experience for users relying on screen readers.
Is it necessary to use useId for every component in a Next.js app?
No, it's not necessary to use useId for every component. It should be used judiciously for components that require unique identifiers, such as forms or dynamic lists where proper identification is crucial.