Next.js is a popular framework for building web applications with React. One of its most handy features is how easily you can implement redirects. Redirects help manage your web traffic, ensuring users end up on the correct page, even if they initially head in the wrong direction. This guide walks you through the basics of Next.js redirects and explores various ways you can use them effectively. Whether you're a beginner or just brushing up on your Next.js skills, this guide is for you.
understanding Next.js redirects
Redirects in Next.js are all about guiding users from one URL to another. This might be necessary for several reasons, such as when a page has moved, a URL has changed, or when you want to direct users based on specific conditions like their location.
what are redirects?
Simply put, a redirect tells your browser to move from one URL to another. It's like telling a friend to meet you at a new pizza place instead of the one that closed down. Redirects can be temporary or permanent, which we'll discuss in more detail.
types of redirects
There are two primary types of redirects in Next.js:
- 301 Redirect (Permanent): This informs search engines and browsers that a page has permanently moved to a new location. Use this when you're sure the original URL will no longer be used.
- 302 Redirect (Temporary): This indicates that a page has moved temporarily. It's like a "be right back" sign for search engines, letting them know the original URL might return later.
implementing redirects in Next.js
Now that you know what redirects are, let's see how you can implement them in Next.js. The good news? It's pretty straightforward.
using the next.config.js file
The file is your go-to place for configuring redirects in Next.js. You can define redirects in this file, and Next.js will handle them automatically.
Here's a simple example:
In this example, any request to will be redirected to , and it's a permanent redirect.
conditional redirects
Sometimes, you might want to redirect users based on certain conditions, like their location or device type. Next.js allows you to set up such conditional redirects using custom logic within the function.
For instance, you can check if a user is on a mobile device and redirect them to a mobile-friendly page:
This example checks the header and redirects users to a mobile version of the site if they're using a mobile device.
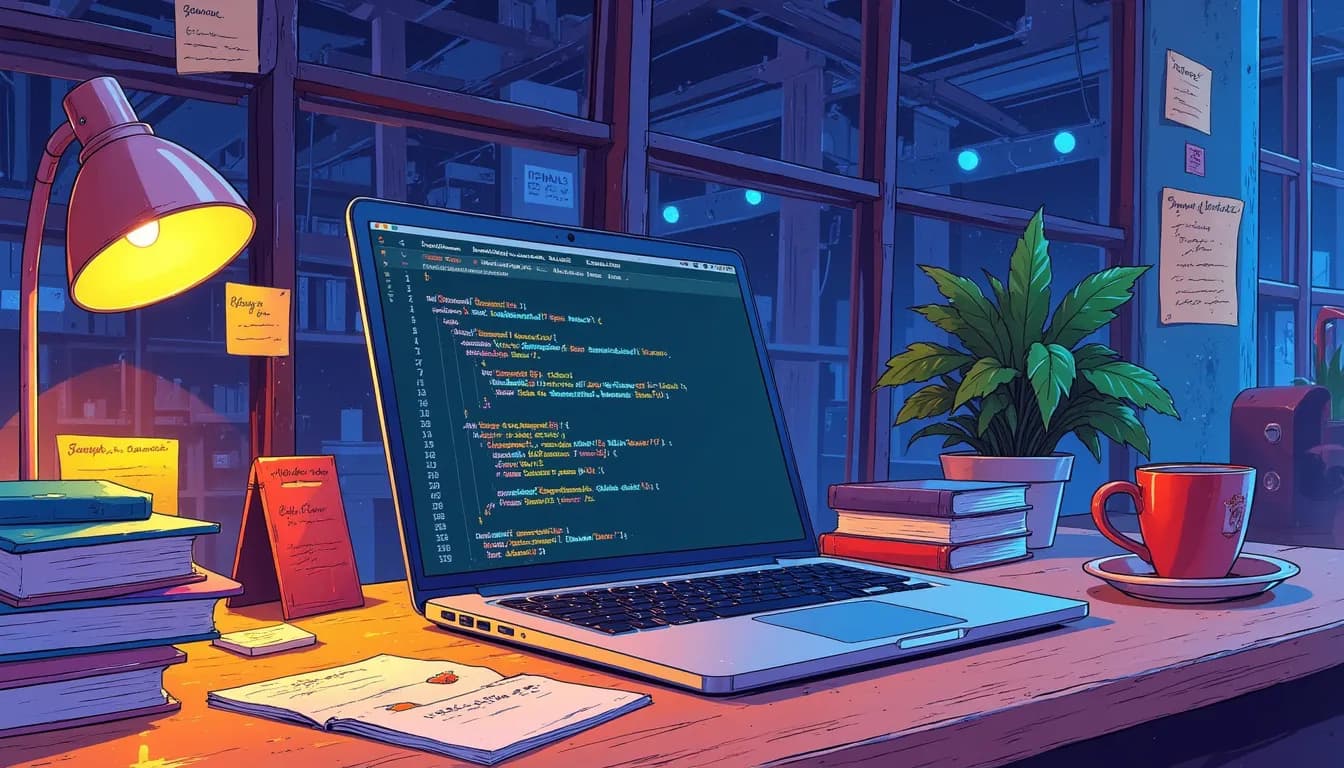
advanced redirect techniques
Next.js offers more advanced redirect techniques that can enhance the user experience and optimize your site.
chained redirects
Chaining redirects is a technique where multiple redirects are configured in sequence. This can be useful when you need to redirect users through several URLs before they reach the final destination.
Here's an example:
In this case, a user navigating to will first be redirected to and then finally to .
redirects with query parameters
Query parameters can be included in your redirects to pass extra information. This is useful for analytics or preserving user data across redirects.
This setup captures a query parameter from the source URL and appends it to the destination URL. So, if a user visits , they'll be redirected to .
best practices for using redirects
Like any tool, redirects are most effective when used wisely. Here are some best practices to keep in mind:
minimize redirect chains
Avoid creating long chains of redirects, as they can slow down the user experience. Try to redirect directly to the final URL whenever possible.
use the correct redirect type
Choose between 301 and 302 redirects based on your needs. If a URL has permanently moved, always opt for a 301 redirect to ensure search engines update their records.
test your redirects
Before deploying changes, test your redirects to ensure they work as expected. You can do this by manually checking URLs or using automated tools.
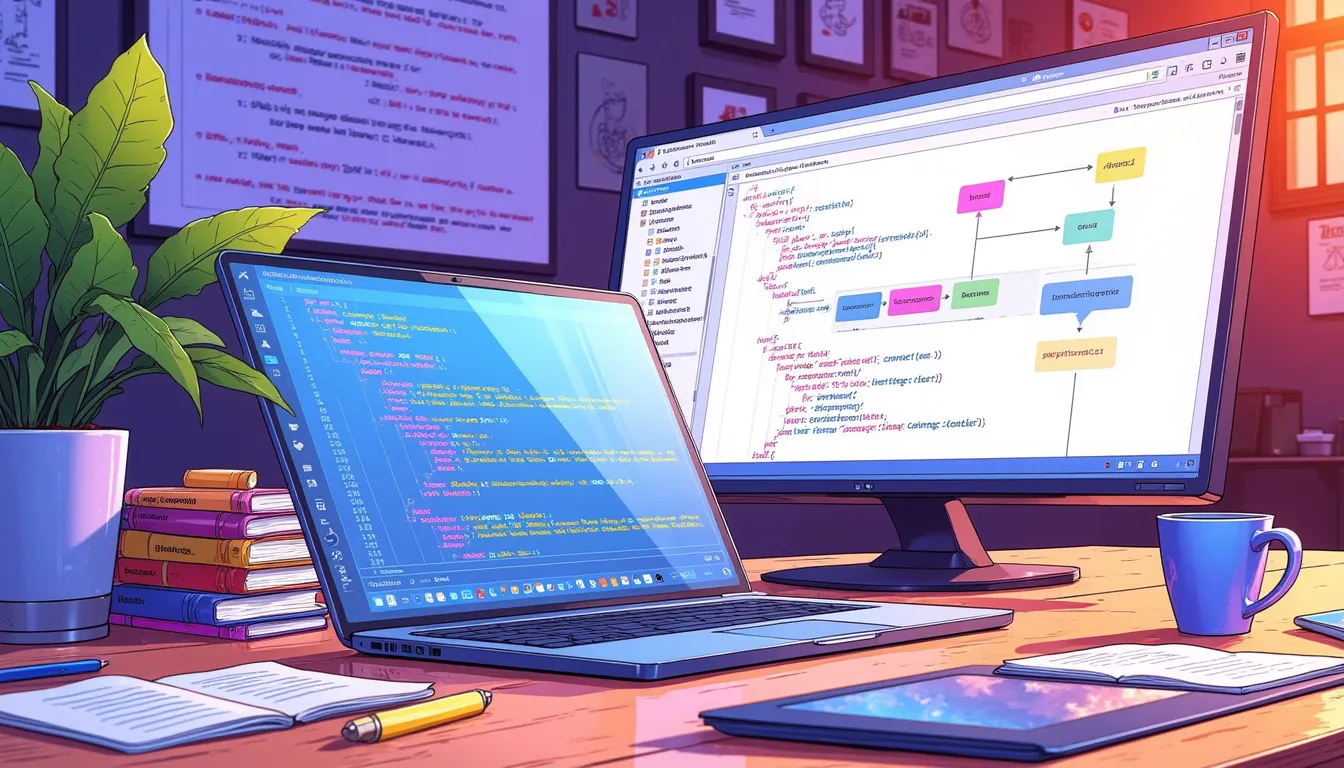
conclusion
Redirects are a powerful feature in Next.js, allowing you to guide users to the right pages while preserving SEO value. By understanding how to set up and manage redirects, you can enhance the usability of your web applications and ensure a smooth user experience. Whether you're implementing simple one-to-one redirects or more complex conditional ones, Next.js makes the process straightforward.
For more detailed information, you can always refer to the official Next.js documentation for updates and best practices. Happy redirecting!
FAQ section
what is a redirect in Next.js?
A redirect in Next.js is a way to send users from one URL to another. It's often used to manage changes in URL structure, move pages, or tailor content delivery based on conditions like user location or device type.
how do I implement a simple redirect in Next.js?
Simple redirects in Next.js are implemented using the file, where you define the source URL and the destination URL. Next.js then handles the redirection automatically.
what is the difference between a 301 and a 302 redirect?
A 301 redirect is permanent and signals to search engines that the original URL has moved for good. A 302 redirect is temporary, suggesting that the original URL might be used again in the future.
can I use query parameters with redirects in Next.js?
Yes, Next.js supports redirects with query parameters, allowing you to pass additional information during the redirect process, which can be useful for analytics or maintaining user session data.
are conditional redirects possible in Next.js?
Yes, Next.js allows you to create conditional redirects using custom logic. This can be implemented by checking parameters like user location or device type and redirecting accordingly.