Learn how React server actions work in Next.js with this easy-to-follow guide. Perfect for developers looking to understand and utilize server actions.
Imagine you're at a fancy restaurant. The server takes your order, goes to the kitchen, and brings your meal back. In web development, server actions work similarly. They take requests, perform tasks on a server, and return results. Simple, right? Today, we're diving into Next.js server actions. By the end of this guide, you'll be serving up web applications like a pro chef.
Next.js, a popular framework for building React applications, has introduced server actions. These help developers handle server-side logic seamlessly. Whether you're a seasoned developer or just starting, understanding server actions can greatly improve how you build your web apps. So grab a cup of coffee, sit back, and let's get started.
What are Next.js server actions?
Server actions in Next.js efficiently handle server-side logic. They enable developers to process data on the server and return it to the client. This is especially useful for data fetching or manipulation, like querying a database or sending an email.
How server actions work
Think of server actions as the waitstaff in our restaurant analogy. They take an order (or request) from the client, perform the necessary actions on the server, and then return a response. This keeps the client (or customer) happy and the app running smoothly.
- Request handling: When a client makes a request, a server action takes over, processes the request, and performs the necessary tasks on the server.
- Data manipulation: Server actions can fetch, update, or delete data based on the client's needs.
- Response delivery: After processing, the server action sends the results back to the client, just like a server bringing your meal.
For more technical details, you can check out the official Next.js documentation here.
Benefits of using server actions
Using server actions in Next.js offers several key benefits:
- Efficiency: By offloading tasks to the server, your app can perform faster and handle more users.
- Security: Sensitive operations, like database queries, are handled on the server, reducing exposure to potential threats.
- Scalability: Server actions make it easier to scale applications, as the heavy lifting is done server-side.
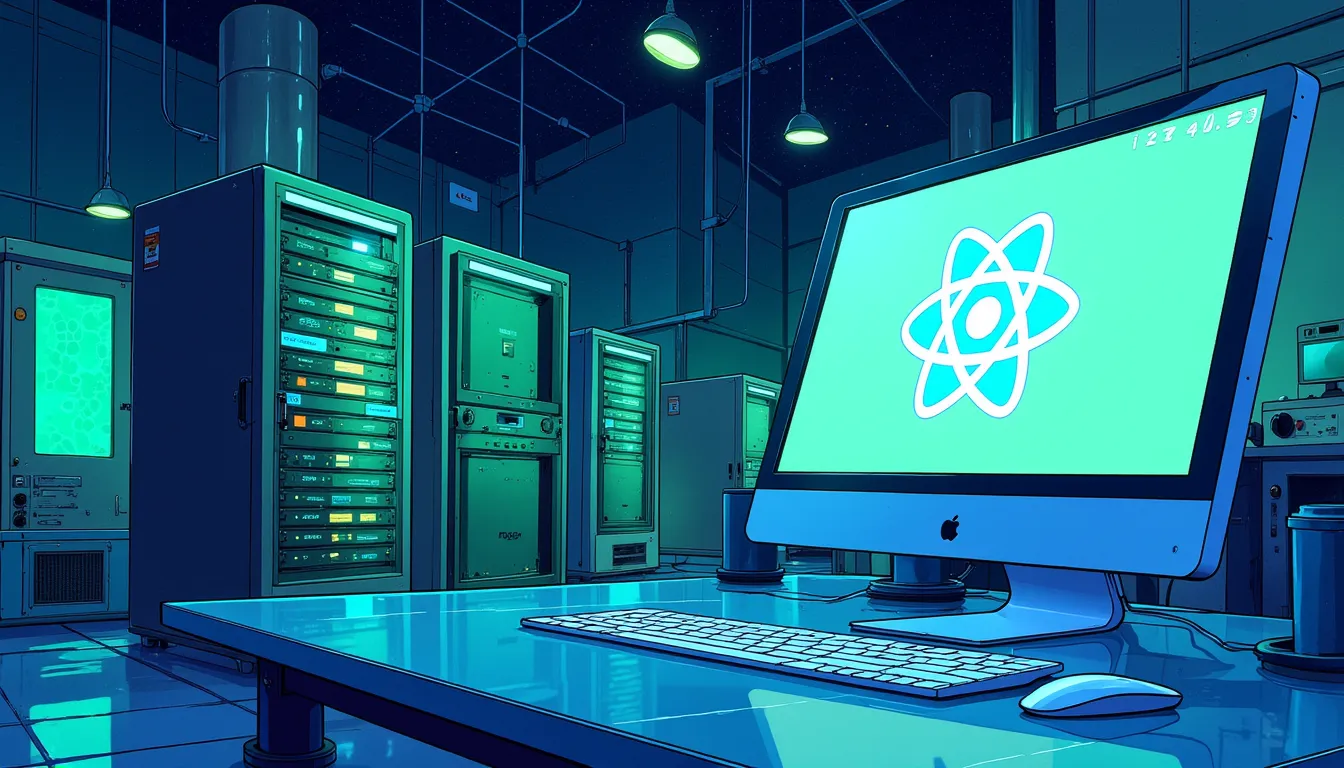
Setting up server actions in your Next.js app
Ready to set up server actions? Let's dive into how to implement them in your Next.js app.
Step 1: Installing Next.js
First, make sure you have Next.js installed. If not, you can easily start a new project by running:
This command sets up a new Next.js application for you. Once that's done, navigate into your project directory.
Step 2: Creating a server action
Now, let's create a simple server action. In Next.js, server actions are typically placed in the directory. Create a new file called in this directory with the following content:
This code sets up a basic server action that responds with a JSON message. It's like saying "hello" from the kitchen!
Step 3: Testing your server action
To test your new server action, start your Next.js development server by running:
Then, visit. You should see your JSON message displayed in the browser. Congrats! You've successfully set up your first server action.
Advanced server actions: Diving deeper
Feeling adventurous? Let's explore some advanced uses of server actions in Next.js.
Data fetching with server actions
Server actions are perfect for fetching data from external APIs or databases. Here's a quick example of how to fetch data from a public API:
This code snippet fetches data from an external API and returns it to the client. It's like the server is fetching ingredients to prepare a delicious dish.
Handling POST requests
Server actions can also handle POST requests, which are useful for operations like saving user data. Here's a simple example:
In this example, the server action greets the user by name, but only if the request method is POST. Otherwise, it returns a "Method not allowed" message.
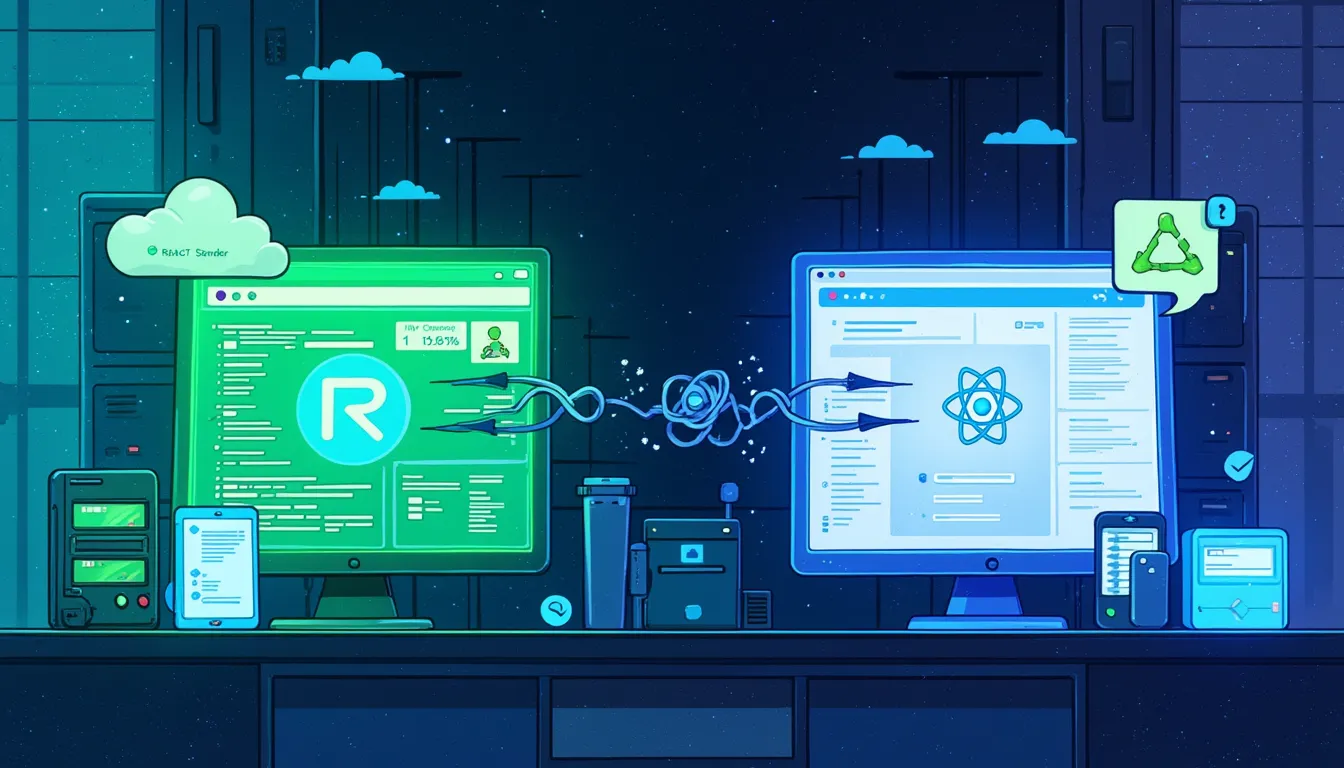
Common pitfalls and troubleshooting
Like any good chef, it's important to know what could go wrong and how to fix it. Let's cover some common pitfalls developers face with server actions.
CORS issues
Cross-Origin Resource Sharing (CORS) can sometimes cause headaches. If your server actions need to communicate with different domains, you'll need to configure CORS properly. Check out the MDN Web Docs for more information on handling CORS issues.
Error handling
Proper error handling is crucial. Imagine a waiter spilling a drink! Make sure to handle errors gracefully in your server actions to provide a smooth user experience. Use blocks and return meaningful error messages.
Performance concerns
Be mindful of performance when using server actions. Offload as much work as possible to the server, but avoid overwhelming it. Consider using caching or optimizing database queries to keep things running smoothly.
Conclusion
And there you have it—a comprehensive guide to understanding and implementing Next.js server actions. From setting up your first server action to tackling advanced scenarios, you've got the tools you need to build efficient, secure, and scalable web applications.
Remember, like any skill, mastering server actions takes practice. So keep experimenting, keep learning, and soon you'll be serving up web apps like a seasoned pro.
For further reading, be sure to explore the official Next.js documentation and keep an eye out for community resources and tutorials. Happy coding!
Frequently asked questions (FAQ) about Next.js server actions
What are server actions in Next.js used for?
Server actions in Next.js are used to handle server-side logic efficiently. They process data on the server and return it to the client, which is particularly useful for operations like data fetching, updating a database, or sending emails.
How do server actions improve application performance?
Server actions improve application performance by offloading processing tasks to the server, which allows the client-side application to run faster and handle more concurrent users effectively.
Can server actions handle POST requests?
Yes, server actions can handle POST requests. They are suitable for operations such as saving user data or processing forms, and can provide customized responses based on the request method.
What are some common pitfalls when using server actions?
Common pitfalls when using server actions include CORS issues, improper error handling, and performance bottlenecks. It's important to configure CORS correctly, handle errors gracefully, and optimize server actions for performance.
How do I get started with server actions in my Next.js app?
To get started with server actions, first ensure that you have Next.js installed, then create server actions by placing necessary logic in designated files within your Next.js project. Tests can be performed by running your development server and accessing the server actions via a browser or API client.